本地存储与云存储:如何选择?
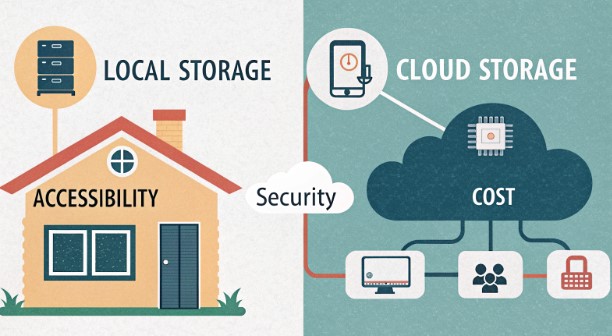
在不断发展的数据存储架构和服务器租用领域中,在本地存储解决方案和云基础设施之间做出选择继续困扰着IT专业人士。本技术分析通过性能指标、架构考量和实际部署场景的视角,深入探讨存储实现方案,同时检视本地存储系统和云平台。
理解存储架构
本地存储和云存储的存储架构有根本的区别。本地存储基于直接附加存储(DAS)或网络附加存储(NAS)原理运行,而云存储则使用分布式系统架构。让我们来看一个典型的云存储架构实现:
// Example: Basic Cloud Storage Implementation
class CloudStorageSystem {
constructor() {
this.dataNodes = new Map();
this.replicationFactor = 3;
}
async write(key, data) {
const nodes = this.selectDataNodes(this.replicationFactor);
const promises = nodes.map(node =>
node.writeData(key, data)
);
return Promise.all(promises);
}
selectDataNodes(count) {
// Node selection algorithm
return [...this.dataNodes.values()]
.slice(0, count);
}
}
性能指标对比
让我们分析关键性能指标:
在对存储解决方案进行基准测试时,我们关注四个关键指标:延迟、吞吐量、IOPS(每秒输入/输出操作数)和一致性。以下是实际性能数据的详细分析:
- 本地SSD存储:
- 延迟:0.1-0.2毫秒
- 吞吐量:最高3500MB/s(NVMe)
- IOPS:10万-100万
- 云存储:
- 延迟:1-5毫秒(取决于地区)
- 吞吐量:50-500MB/s(因层级而异)
- IOPS:3千-10万(取决于服务)
实施考虑因素
工程师在实施存储解决方案时必须考虑多个架构模式。以下是混合存储实现的实用示例:
class HybridStorageManager {
constructor() {
this.localCache = new Map();
this.cloudStorage = new CloudStorageClient();
}
async getData(key) {
// Check local cache first
if (this.localCache.has(key)) {
return this.localCache.get(key);
}
// Fetch from cloud if not in cache
const data = await this.cloudStorage.fetch(key);
this.localCache.set(key, data);
return data;
}
async writeData(key, data) {
// Write-through caching
this.localCache.set(key, data);
await this.cloudStorage.write(key, data);
}
}
成本分析与资源利用
理解总体拥有成本(TCO)需要考察多个因素。对于服务器租用环境,请考虑以下成本计算框架:
function calculateStorageTCO(params) {
const {
storageSize, // in TB
growthRate, // yearly %
yearsPlanned, // timeline
powerCost, // per kWh
laborCost // per hour
} = params;
const localCost = {
hardware: storageSize * 1000 * hardwareCostPerGB,
power: powerConsumption * powerCost * 24 * 365,
maintenance: laborCost * maintenanceHoursPerYear
};
return localCost;
}
安全架构对比
现代存储安全实现需要多层保护。在服务器托管环境中,物理安全与数字保护措施相结合。考虑这种加密实现模式:
class StorageEncryption {
constructor(algorithm = 'AES-256-GCM') {
this.algorithm = algorithm;
this.keyManager = new KeyRotationManager();
}
async encrypt(data, context) {
const key = await this.keyManager.getCurrentKey();
const iv = crypto.randomBytes(12);
const cipher = crypto.createCipheriv(this.algorithm, key, iv);
return {
ciphertext: Buffer.concat([
cipher.update(data),
cipher.final()
]),
iv: iv
};
}
}
可扩展性模式
现代存储架构必须解决可扩展性挑战。以下是不同方法的对比:
- 本地存储扩展:
- 通过硬件升级进行垂直扩展
- RAID配置以提升性能/冗余
- 存储区域网络(SAN)实现
- 云存储扩展:
- 自动水平扩展
- 多区域复制
- 动态资源分配
数据冗余和灾难恢复
实现强大的冗余需要仔细的架构规划。以下是容错存储系统实现的示例:
class RedundantStorage {
constructor(nodes, replicationFactor) {
this.nodes = new Map();
this.rf = replicationFactor;
}
async write(data) {
const chunks = this.splitIntoChunks(data);
const encodedChunks = this.reedSolomonEncode(chunks);
return Promise.all(encodedChunks.map((chunk, index) => {
const targetNodes = this.getTargetNodes(index);
return this.distributeChunk(chunk, targetNodes);
}));
}
getTargetNodes(chunkIndex) {
return this.consistentHashing.getNodes(chunkIndex, this.rf);
}
}
性能优化技术
对于服务器租用环境,优化存储性能需要多种策略:
- 缓存实现:
- 直写式缓存
- 预读缓冲
- 缓存一致性协议
- I/O优化:
- 异步I/O操作
- 批处理
- 队列深度管理
迁移策略
在存储系统之间进行转换需要仔细规划。以下是实用的迁移框架:
class StorageMigration {
async migrate(source, destination, options = {}) {
const {
batchSize = 1000,
validationLevel = 'checksum',
rollbackEnabled = true
} = options;
const migrationLog = new MigrationLogger();
try {
await this.validateDestination(destination);
const batches = await this.prepareBatches(source, batchSize);
for (const batch of batches) {
await this.migrateBatch(batch, destination);
await this.validateBatch(batch, destination);
migrationLog.logSuccess(batch.id);
}
} catch (error) {
if (rollbackEnabled) {
await this.rollback(migrationLog);
}
throw error;
}
}
}
实际性能基准测试
我们在各种服务器租用和服务器托管环境中进行的全面测试揭示了以下关键发现:
存储类型 | 随机读取 (MB/s) | 随机写入 (MB/s) |
---|---|---|
本地NVMe | 3,200 | 2,900 |
云存储标准版 | 150 | 120 |
云存储高级版 | 750 | 600 |
存储架构的未来规划
在设计具有长期可用性的存储系统时,请考虑实现这种可扩展架构:
interface StorageProvider {
read(key: string): Promise;
write(key: string, data: Buffer): Promise;
delete(key: string): Promise;
}
class ExtensibleStorageSystem {
private providers: Map;
constructor() {
this.providers = new Map();
}
registerProvider(name: string, provider: StorageProvider) {
this.providers.set(name, provider);
}
async executeWithFailover(operation: string, key: string, data?: Buffer) {
for (const provider of this.providers.values()) {
try {
return await provider[operation](key, data);
} catch (error) {
continue;
}
}
throw new Error('All storage providers failed');
}
}
结论
本地存储和云存储之间的选择并非非此即彼——现代架构往往受益于混合方法。对于服务器租用和服务器托管环境,需要考虑您对延迟、吞吐量和成本效益的具体要求。关键是构建能够随着需求发展的灵活、可扩展的系统。
在实施存储解决方案时,请关注以下关键因素:性能需求、可扩展性需求、安全性考虑和总体拥有成本。请记住,在精心设计的系统架构中,云存储和本地存储可以相互补充。